Chapter 7: Nonlinear Valuation#
rs_utility.jl#
include("s_approx.jl")
using LinearAlgebra, QuantEcon
function create_rs_utility_model(;
n=180, # size of state space
β=0.95, # time discount factor
ρ=0.96, # correlation coef in AR(1)
σ=0.1, # volatility
θ=-1.0) # risk aversion
mc = tauchen(n, ρ, σ, 0, 10) # n_std = 10
x_vals, P = mc.state_values, mc.p
r = x_vals # special case u(c(x)) = x
return (; β, θ, ρ, σ, r, x_vals, P)
end
function K(v, model)
(; β, θ, ρ, σ, r, x_vals, P) = model
return r + (β/θ) * log.(P * (exp.(θ*v)))
end
function compute_rs_utility(model)
(; β, θ, ρ, σ, r, x_vals, P) = model
v_init = zeros(length(x_vals))
v_star = successive_approx(v -> K(v, model),
v_init, tolerance=1e-10)
return v_star
end
# Plots
using PyPlot
using LaTeXStrings
PyPlot.matplotlib[:rc]("text", usetex=true) # allow tex rendering
fontsize=16
function plot_v(; savefig=false,
figname="./figures/rs_utility_1.pdf")
fig, ax = plt.subplots(figsize=(10, 5.2))
model = create_rs_utility_model()
(; β, θ, ρ, σ, r, x_vals, P) = model
a = 1/(1 - (ρ*β))
b = (β /(1 - β)) * (θ/2) * (a*σ)^2
v_star = compute_rs_utility(model)
v_star_a = a * x_vals .+ b
ax.plot(x_vals, v_star, lw=2, alpha=0.7, label="approximate fixed point")
ax.plot(x_vals, v_star_a, "k--", lw=2, alpha=0.7, label=L"v(x)=ax + b")
ax.set_xlabel(L"x", fontsize=fontsize)
ax.legend(frameon=false, fontsize=fontsize, loc="upper left")
if savefig
fig.savefig(figname)
end
end
function plot_multiple_v(; savefig=false,
figname="./figures/rs_utility_2.pdf")
fig, ax = plt.subplots(figsize=(10, 5.2))
σ_vals = 0.05, 0.1
for σ in σ_vals
model = create_rs_utility_model(σ=σ)
(; β, θ, r, x_vals, P) = model
v_star = compute_rs_utility(model)
ax.plot(x_vals, v_star, lw=2, alpha=0.7, label=L"\sigma="*"$σ")
ax.set_xlabel(L"x", fontsize=fontsize)
ax.set_ylabel(L"v(x)", fontsize=fontsize)
end
ax.legend(frameon=false, fontsize=fontsize, loc="upper left")
if savefig
fig.savefig(figname)
end
end
plot_multiple_v (generic function with 1 method)
plot_v(savefig=true)
Completed iteration 25 with error 0.6551733102188564.
Completed iteration 50 with error 0.16567516808198945.
Completed iteration 75 with error 0.044889308777882775.
Completed iteration 100 with error 0.012367970868957912.
Completed iteration 125 with error 0.0034238793852026106.
Completed iteration 150 with error 0.0009491803696306533.
Completed iteration 175 with error 0.00026324562215052083.
Completed iteration 200 with error 7.301768051348745e-5.
Completed iteration 225 with error 2.025401865068943e-5.
Completed iteration 250 with error 5.6182266447990514e-6.
Completed iteration 275 with error 1.5584352581754501e-6.
Completed iteration 300 with error 4.322935041045639e-7.
Completed iteration 325 with error 1.1991370030273174e-7.
Completed iteration 350 with error 3.326281472482151e-8.
Completed iteration 375 with error 9.226766906067496e-9.
Completed iteration 400 with error 2.559410461344669e-9.
Completed iteration 425 with error 7.099600907167769e-10.
Completed iteration 450 with error 1.9694113007062697e-10.
Terminated successfully in 465 iterations.
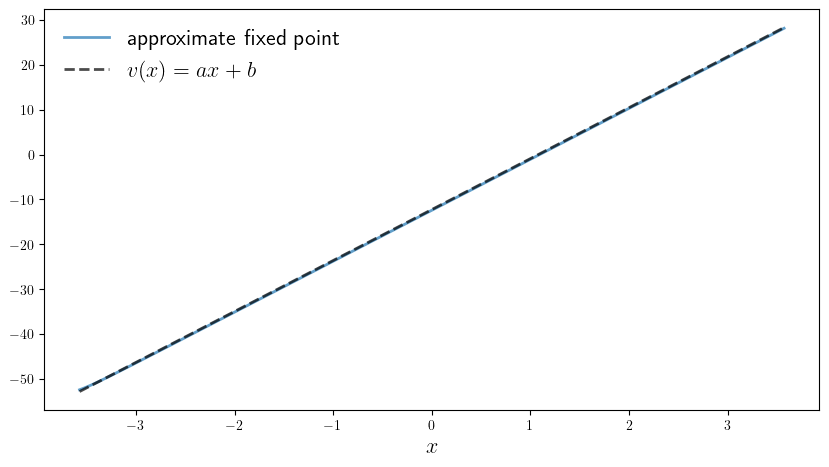
plot_multiple_v(savefig=true)
Completed iteration 25 with error 0.2636239967879277.
Completed iteration 50 with error 0.05190477488617873.
Completed iteration 75 with error 0.012350547797844058.
Completed iteration 100 with error 0.003222014685647423.
Completed iteration 125 with error 0.0008733772549120999.
Completed iteration 150 with error 0.00024022882517016342.
Completed iteration 175 with error 6.643334072009566e-5.
Completed iteration 200 with error 1.840755902904334e-5.
Completed iteration 225 with error 5.104029845881541e-6.
Completed iteration 250 with error 1.4156012113630823e-6.
Completed iteration 275 with error 3.926526765951621e-7.
Completed iteration 300 with error 1.0891572443938458e-7.
Completed iteration 325 with error 3.021188277330111e-8.
Completed iteration 350 with error 8.38044300621732e-9.
Completed iteration 375 with error 2.3246471414495318e-9.
Completed iteration 400 with error 6.448388489843637e-10.
Completed iteration 425 with error 1.7887202830024762e-10.
Terminated successfully in 438 iterations.
Completed iteration 25 with error 0.6551733102188564.
Completed iteration 50 with error 0.16567516808198945.
Completed iteration 75 with error 0.044889308777882775.
Completed iteration 100 with error 0.012367970868957912.
Completed iteration 125 with error 0.0034238793852026106.
Completed iteration 150 with error 0.0009491803696306533.
Completed iteration 175 with error 0.00026324562215052083.
Completed iteration 200 with error 7.301768051348745e-5.
Completed iteration 225 with error 2.025401865068943e-5.
Completed iteration 250 with error 5.6182266447990514e-6.
Completed iteration 275 with error 1.5584352581754501e-6.
Completed iteration 300 with error 4.322935041045639e-7.
Completed iteration 325 with error 1.1991370030273174e-7.
Completed iteration 350 with error 3.326281472482151e-8.
Completed iteration 375 with error 9.226766906067496e-9.
Completed iteration 400 with error 2.559410461344669e-9.
Completed iteration 425 with error 7.099600907167769e-10.
Completed iteration 450 with error 1.9694113007062697e-10.
Terminated successfully in 465 iterations.
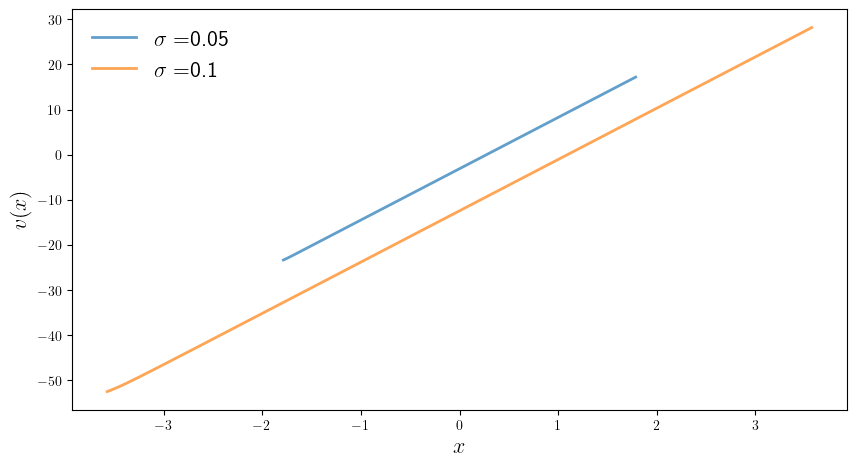
ez_utility.jl#
"""
Epstein--Zin utility: solving the recursion for a given consumption
path.
"""
include("s_approx.jl")
using LinearAlgebra, QuantEcon
function create_ez_utility_model(;
n=200, # size of state space
ρ=0.96, # correlation coef in AR(1)
σ=0.1, # volatility
β=0.99, # time discount factor
α=0.75, # EIS parameter
γ=-2.0) # risk aversion parameter
mc = tauchen(n, ρ, σ, 0, 5)
x_vals, P = mc.state_values, mc.p
c = exp.(x_vals)
return (; β, ρ, σ, α, γ, c, x_vals, P)
end
function K(v, model)
(; β, ρ, σ, α, γ, c, x_vals, P) = model
R = (P * (v.^γ)).^(1/γ)
return ((1 - β) * c.^α + β * R.^α).^(1/α)
end
function compute_ez_utility(model)
v_init = ones(length(model.x_vals))
v_star = successive_approx(v -> K(v, model),
v_init,
tolerance=1e-10)
return v_star
end
# Plots
using PyPlot
using LaTeXStrings
PyPlot.matplotlib[:rc]("text", usetex=true) # allow tex rendering
fontsize=16
function plot_convergence(; savefig=false,
num_iter=100,
figname="./figures/ez_utility_c.pdf")
fig, ax = plt.subplots(figsize=(10, 5.2))
model = create_ez_utility_model()
(; β, ρ, σ, α, γ, c, x_vals, P) = model
v_star = compute_ez_utility(model)
v = 0.1 * v_star
ax.plot(x_vals, v, lw=3, "k-", alpha=0.7, label=L"v_0")
greys = [string(g) for g in LinRange(0.0, 0.4, num_iter)]
greys = reverse(greys)
for (i, g) in enumerate(greys)
ax.plot(x_vals, v, "k-", color=g, lw=1, alpha=0.7)
for t in 1:20
v = K(v, model)
end
end
v_star = compute_ez_utility(model)
ax.plot(x_vals, v_star, lw=3, alpha=0.7, label=L"v^*")
ax.set_xlabel(L"x", fontsize=fontsize)
ax.legend(frameon=false, fontsize=fontsize, loc="upper left")
if savefig
fig.savefig(figname)
end
end
function plot_v(; savefig=false,
figname="./figures/ez_utility_1.pdf")
fig, ax = plt.subplots(figsize=(10, 5.2))
model = create_ez_utility_model()
(; β, ρ, σ, α, γ, c, x_vals, P) = model
v_star = compute_ez_utility(model)
ax.plot(x_vals, v_star, lw=2, alpha=0.7, label=L"v^*")
ax.set_xlabel(L"x", fontsize=fontsize)
ax.legend(frameon=false, fontsize=fontsize, loc="upper left")
if savefig
fig.savefig(figname)
end
end
function vary_gamma(; gamma_vals=[1.0, -8.0],
savefig=false,
figname="./figures/ez_utility_2.pdf")
fig, ax = plt.subplots(figsize=(10, 5.2))
for γ in gamma_vals
model = create_ez_utility_model(γ=γ)
(; β, ρ, σ, α, γ, c, x_vals, P) = model
v_star = compute_ez_utility(model)
ax.plot(x_vals, v_star, lw=2, alpha=0.7, label=L"\gamma="*"$γ")
ax.set_xlabel(L"x", fontsize=fontsize)
ax.set_ylabel(L"v(x)", fontsize=fontsize)
end
ax.legend(frameon=false, fontsize=fontsize, loc="upper left")
if savefig
fig.savefig(figname)
end
end
function vary_alpha(; alpha_vals=[0.5, 0.6],
savefig=false,
figname="./figures/ez_utility_3.pdf")
fig, ax = plt.subplots(figsize=(10, 5.2))
for α in alpha_vals
model = create_ez_utility_model(α=α)
(; β, ρ, σ, α, γ, c, x_vals, P) = model
v_star = compute_ez_utility(model)
ax.plot(x_vals, v_star, lw=2, alpha=0.7, label=L"\alpha="*"$α")
ax.set_xlabel(L"x", fontsize=fontsize)
ax.set_ylabel(L"v(x)", fontsize=fontsize)
end
ax.legend(frameon=false, fontsize=fontsize, loc="upper left")
if savefig
fig.savefig(figname)
end
end
vary_alpha (generic function with 1 method)
plot_convergence(savefig=true)
Completed iteration 25 with error 0.007206209255429918.
Completed iteration 50 with error 0.001567197885917082.
Completed iteration 75 with error 0.0004273965382449729.
Completed iteration 100 with error 0.00017898582383268913.
Completed iteration 125 with error 9.707282676585383e-5.
Completed iteration 150 with error 6.428406156233635e-5.
Completed iteration 175 with error 5.310395754820618e-5.
Completed iteration 200 with error 4.2962945760338656e-5.
Completed iteration 225 with error 3.4085282641926895e-5.
Completed iteration 250 with error 2.6864322490993686e-5.
Completed iteration 275 with error 2.112547014143651e-5.
Completed iteration 300 with error 1.659984383839408e-5.
Completed iteration 325 with error 1.3040400105968075e-5.
Completed iteration 350 with error 1.02433780719835e-5.
Completed iteration 375 with error 8.046119378546379e-6.
Completed iteration 400 with error 6.320173207674529e-6.
Completed iteration 425 with error 4.964472275492682e-6.
Completed iteration 450 with error 3.899592445177902e-6.
Completed iteration 475 with error 3.0631425254057376e-6.
Completed iteration 500 with error 2.4061169785483116e-6.
Completed iteration 525 with error 1.8900248719422308e-6.
Completed iteration 550 with error 1.4846336273688365e-6.
Completed iteration 575 with error 1.1661968122300692e-6.
Completed iteration 600 with error 9.16062327993572e-7.
Completed iteration 625 with error 7.195793338965473e-7.
Completed iteration 650 with error 5.652397836453105e-7.
Completed iteration 675 with error 4.4400418119927565e-7.
Completed iteration 700 with error 3.487720177108855e-7.
Completed iteration 725 with error 2.7396582580330175e-7.
Completed iteration 750 with error 2.152044744629933e-7.
Completed iteration 775 with error 1.690465507575567e-7.
Completed iteration 800 with error 1.3278879240630204e-7.
Completed iteration 825 with error 1.0430775509995271e-7.
Completed iteration 850 with error 8.193544798196228e-8.
Completed iteration 875 with error 6.436164090573016e-8.
Completed iteration 900 with error 5.055713092616543e-8.
Completed iteration 925 with error 3.9713462740564864e-8.
Completed iteration 950 with error 3.119558367181696e-8.
Completed iteration 975 with error 2.4504649198675565e-8.
Completed iteration 1000 with error 1.9248809657312904e-8.
Completed iteration 1025 with error 1.5120260599132962e-8.
Completed iteration 1050 with error 1.1877217209743662e-8.
Completed iteration 1075 with error 9.32975185996554e-9.
Completed iteration 1100 with error 7.328675666329332e-9.
Completed iteration 1125 with error 5.7567970390692835e-9.
Completed iteration 1150 with error 4.522060503830971e-9.
Completed iteration 1175 with error 3.55215368230688e-9.
Completed iteration 1200 with error 2.790276454334162e-9.
Completed iteration 1225 with error 2.191808512463922e-9.
Completed iteration 1250 with error 1.7217023362547934e-9.
Completed iteration 1275 with error 1.3524261710529117e-9.
Completed iteration 1300 with error 1.0623533164277887e-9.
Completed iteration 1325 with error 8.344962498796349e-10.
Completed iteration 1350 with error 6.555103126970607e-10.
Completed iteration 1375 with error 5.149147774829999e-10.
Completed iteration 1400 with error 4.044742318853878e-10.
Completed iteration 1425 with error 3.177211826965731e-10.
Completed iteration 1450 with error 2.495754714004761e-10.
Completed iteration 1475 with error 1.9604562417896432e-10.
Completed iteration 1500 with error 1.5399703734431114e-10.
Completed iteration 1525 with error 1.2096723622789796e-10.
Terminated successfully in 1546 iterations.
Completed iteration 25 with error 0.007206209255429918.
Completed iteration 50 with error 0.001567197885917082.
Completed iteration 75 with error 0.0004273965382449729.
Completed iteration 100 with error 0.00017898582383268913.
Completed iteration 125 with error 9.707282676585383e-5.
Completed iteration 150 with error 6.428406156233635e-5.
Completed iteration 175 with error 5.310395754820618e-5.
Completed iteration 200 with error 4.2962945760338656e-5.
Completed iteration 225 with error 3.4085282641926895e-5.
Completed iteration 250 with error 2.6864322490993686e-5.
Completed iteration 275 with error 2.112547014143651e-5.
Completed iteration 300 with error 1.659984383839408e-5.
Completed iteration 325 with error 1.3040400105968075e-5.
Completed iteration 350 with error 1.02433780719835e-5.
Completed iteration 375 with error 8.046119378546379e-6.
Completed iteration 400 with error 6.320173207674529e-6.
Completed iteration 425 with error 4.964472275492682e-6.
Completed iteration 450 with error 3.899592445177902e-6.
Completed iteration 475 with error 3.0631425254057376e-6.
Completed iteration 500 with error 2.4061169785483116e-6.
Completed iteration 525 with error 1.8900248719422308e-6.
Completed iteration 550 with error 1.4846336273688365e-6.
Completed iteration 575 with error 1.1661968122300692e-6.
Completed iteration 600 with error 9.16062327993572e-7.
Completed iteration 625 with error 7.195793338965473e-7.
Completed iteration 650 with error 5.652397836453105e-7.
Completed iteration 675 with error 4.4400418119927565e-7.
Completed iteration 700 with error 3.487720177108855e-7.
Completed iteration 725 with error 2.7396582580330175e-7.
Completed iteration 750 with error 2.152044744629933e-7.
Completed iteration 775 with error 1.690465507575567e-7.
Completed iteration 800 with error 1.3278879240630204e-7.
Completed iteration 825 with error 1.0430775509995271e-7.
Completed iteration 850 with error 8.193544798196228e-8.
Completed iteration 875 with error 6.436164090573016e-8.
Completed iteration 900 with error 5.055713092616543e-8.
Completed iteration 925 with error 3.9713462740564864e-8.
Completed iteration 950 with error 3.119558367181696e-8.
Completed iteration 975 with error 2.4504649198675565e-8.
Completed iteration 1000 with error 1.9248809657312904e-8.
Completed iteration 1025 with error 1.5120260599132962e-8.
Completed iteration 1050 with error 1.1877217209743662e-8.
Completed iteration 1075 with error 9.32975185996554e-9.
Completed iteration 1100 with error 7.328675666329332e-9.
Completed iteration 1125 with error 5.7567970390692835e-9.
Completed iteration 1150 with error 4.522060503830971e-9.
Completed iteration 1175 with error 3.55215368230688e-9.
Completed iteration 1200 with error 2.790276454334162e-9.
Completed iteration 1225 with error 2.191808512463922e-9.
Completed iteration 1250 with error 1.7217023362547934e-9.
Completed iteration 1275 with error 1.3524261710529117e-9.
Completed iteration 1300 with error 1.0623533164277887e-9.
Completed iteration 1325 with error 8.344962498796349e-10.
Completed iteration 1350 with error 6.555103126970607e-10.
Completed iteration 1375 with error 5.149147774829999e-10.
Completed iteration 1400 with error 4.044742318853878e-10.
Completed iteration 1425 with error 3.177211826965731e-10.
Completed iteration 1450 with error 2.495754714004761e-10.
Completed iteration 1475 with error 1.9604562417896432e-10.
Completed iteration 1500 with error 1.5399703734431114e-10.
Completed iteration 1525 with error 1.2096723622789796e-10.
Terminated successfully in 1546 iterations.
sys:1: UserWarning: color is redundantly defined by the 'color' keyword argument and the fmt string "k-" (-> color='k'). The keyword argument will take precedence.
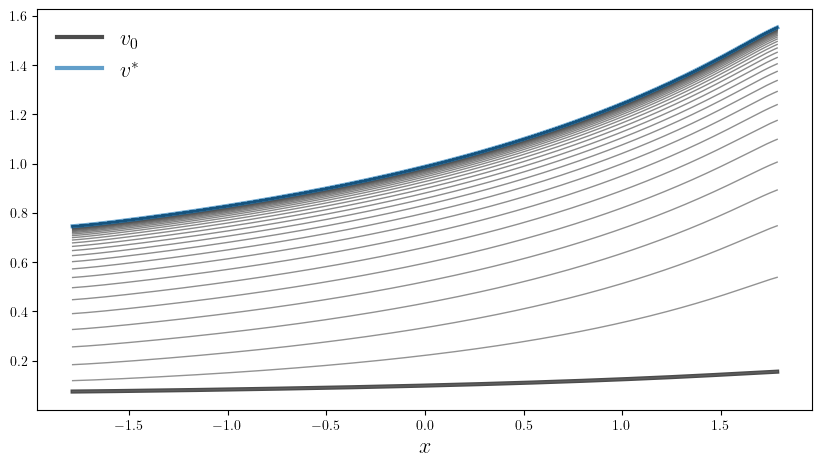
plot_v(savefig=true)
Completed iteration 25 with error 0.007206209255429918.
Completed iteration 50 with error 0.001567197885917082.
Completed iteration 75 with error 0.0004273965382449729.
Completed iteration 100 with error 0.00017898582383268913.
Completed iteration 125 with error 9.707282676585383e-5.
Completed iteration 150 with error 6.428406156233635e-5.
Completed iteration 175 with error 5.310395754820618e-5.
Completed iteration 200 with error 4.2962945760338656e-5.
Completed iteration 225 with error 3.4085282641926895e-5.
Completed iteration 250 with error 2.6864322490993686e-5.
Completed iteration 275 with error 2.112547014143651e-5.
Completed iteration 300 with error 1.659984383839408e-5.
Completed iteration 325 with error 1.3040400105968075e-5.
Completed iteration 350 with error 1.02433780719835e-5.
Completed iteration 375 with error 8.046119378546379e-6.
Completed iteration 400 with error 6.320173207674529e-6.
Completed iteration 425 with error 4.964472275492682e-6.
Completed iteration 450 with error 3.899592445177902e-6.
Completed iteration 475 with error 3.0631425254057376e-6.
Completed iteration 500 with error 2.4061169785483116e-6.
Completed iteration 525 with error 1.8900248719422308e-6.
Completed iteration 550 with error 1.4846336273688365e-6.
Completed iteration 575 with error 1.1661968122300692e-6.
Completed iteration 600 with error 9.16062327993572e-7.
Completed iteration 625 with error 7.195793338965473e-7.
Completed iteration 650 with error 5.652397836453105e-7.
Completed iteration 675 with error 4.4400418119927565e-7.
Completed iteration 700 with error 3.487720177108855e-7.
Completed iteration 725 with error 2.7396582580330175e-7.
Completed iteration 750 with error 2.152044744629933e-7.
Completed iteration 775 with error 1.690465507575567e-7.
Completed iteration 800 with error 1.3278879240630204e-7.
Completed iteration 825 with error 1.0430775509995271e-7.
Completed iteration 850 with error 8.193544798196228e-8.
Completed iteration 875 with error 6.436164090573016e-8.
Completed iteration 900 with error 5.055713092616543e-8.
Completed iteration 925 with error 3.9713462740564864e-8.
Completed iteration 950 with error 3.119558367181696e-8.
Completed iteration 975 with error 2.4504649198675565e-8.
Completed iteration 1000 with error 1.9248809657312904e-8.
Completed iteration 1025 with error 1.5120260599132962e-8.
Completed iteration 1050 with error 1.1877217209743662e-8.
Completed iteration 1075 with error 9.32975185996554e-9.
Completed iteration 1100 with error 7.328675666329332e-9.
Completed iteration 1125 with error 5.7567970390692835e-9.
Completed iteration 1150 with error 4.522060503830971e-9.
Completed iteration 1175 with error 3.55215368230688e-9.
Completed iteration 1200 with error 2.790276454334162e-9.
Completed iteration 1225 with error 2.191808512463922e-9.
Completed iteration 1250 with error 1.7217023362547934e-9.
Completed iteration 1275 with error 1.3524261710529117e-9.
Completed iteration 1300 with error 1.0623533164277887e-9.
Completed iteration 1325 with error 8.344962498796349e-10.
Completed iteration 1350 with error 6.555103126970607e-10.
Completed iteration 1375 with error 5.149147774829999e-10.
Completed iteration 1400 with error 4.044742318853878e-10.
Completed iteration 1425 with error 3.177211826965731e-10.
Completed iteration 1450 with error 2.495754714004761e-10.
Completed iteration 1475 with error 1.9604562417896432e-10.
Completed iteration 1500 with error 1.5399703734431114e-10.
Completed iteration 1525 with error 1.2096723622789796e-10.
Terminated successfully in 1546 iterations.
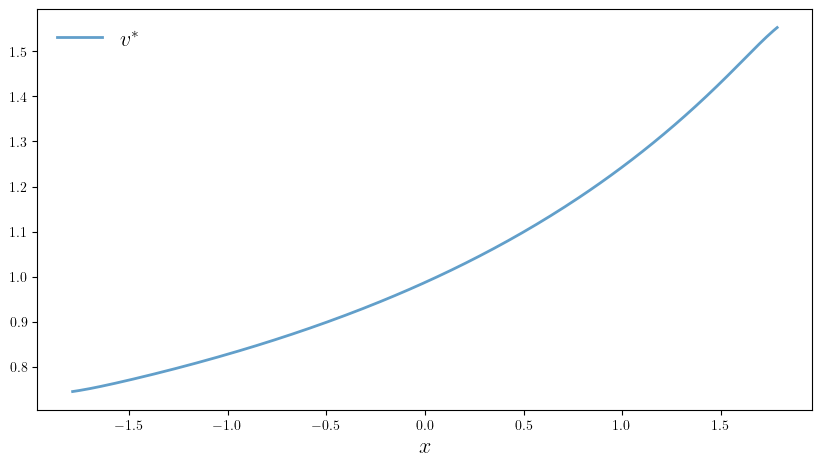
vary_gamma(savefig=true)
Completed iteration 25 with error 0.0079928250990704.
Completed iteration 50 with error 0.0022236699030695473.
Completed iteration 75 with error 0.0007925233675871723.
Completed iteration 100 with error 0.00037000891531158153.
Completed iteration 125 with error 0.00022014006478499049.
Completed iteration 150 with error 0.000152329026353204.
Completed iteration 175 with error 0.00011314688364394421.
Completed iteration 200 with error 8.647303897624248e-5.
Completed iteration 225 with error 6.67984175424241e-5.
Completed iteration 250 with error 5.180167311036321e-5.
Completed iteration 275 with error 4.0227726982866585e-5.
Completed iteration 300 with error 3.1254842565831e-5.
Completed iteration 325 with error 2.4287249895671437e-5.
Completed iteration 350 with error 1.887378865372824e-5.
Completed iteration 375 with error 1.4667052229189181e-5.
Completed iteration 400 with error 1.1397890212316852e-5.
Completed iteration 425 with error 8.857330340950043e-6.
Completed iteration 450 with error 6.883005259750163e-6.
Completed iteration 475 with error 5.348730677257052e-6.
Completed iteration 500 with error 4.1564373636227e-6.
Completed iteration 525 with error 3.229907427737544e-6.
Completed iteration 550 with error 2.509906823489061e-6.
Completed iteration 575 with error 1.9504018531346645e-6.
Completed iteration 600 with error 1.5156181834008464e-6.
Completed iteration 625 with error 1.1777548305502705e-6.
Completed iteration 650 with error 9.152073530138694e-7.
Completed iteration 675 with error 7.111868745024452e-7.
Completed iteration 700 with error 5.526468198624457e-7.
Completed iteration 725 with error 4.2944879763417987e-7.
Completed iteration 750 with error 3.3371438834528533e-7.
Completed iteration 775 with error 2.593213874746425e-7.
Completed iteration 800 with error 2.0151233459664297e-7.
Completed iteration 825 with error 1.5659029850567663e-7.
Completed iteration 850 with error 1.2168246632526802e-7.
Completed iteration 875 with error 9.455643867894992e-8.
Completed iteration 900 with error 7.347746588948212e-8.
Completed iteration 925 with error 5.7097514716275555e-8.
Completed iteration 950 with error 4.43690586493517e-8.
Completed iteration 975 with error 3.447809060475038e-8.
Completed iteration 1000 with error 2.6792065010994293e-8.
Completed iteration 1025 with error 2.0819445500919187e-8.
Completed iteration 1050 with error 1.6178271167177627e-8.
Completed iteration 1075 with error 1.257173076751883e-8.
Completed iteration 1100 with error 9.769177911067572e-9.
Completed iteration 1125 with error 7.591383965888099e-9.
Completed iteration 1150 with error 5.8990745621656515e-9.
Completed iteration 1175 with error 4.584023827192141e-9.
Completed iteration 1200 with error 3.5621299243615567e-9.
Completed iteration 1225 with error 2.768042017819994e-9.
Completed iteration 1250 with error 2.150976063930443e-9.
Completed iteration 1275 with error 1.6714698514164184e-9.
Completed iteration 1300 with error 1.298857466025538e-9.
Completed iteration 1325 with error 1.009309302801853e-9.
Completed iteration 1350 with error 7.843090621406645e-10.
Completed iteration 1375 with error 6.094669213752013e-10.
Completed iteration 1400 with error 4.736018244244633e-10.
Completed iteration 1425 with error 3.680240556747094e-10.
Completed iteration 1450 with error 2.85982570957799e-10.
Completed iteration 1475 with error 2.2222956808093386e-10.
Completed iteration 1500 with error 1.726889742315052e-10.
Completed iteration 1525 with error 1.3419243494183775e-10.
Completed iteration 1550 with error 1.042774755433129e-10.
Terminated successfully in 1556 iterations.
Completed iteration 25 with error 0.005805024318952023.
Completed iteration 50 with error 0.0016587166980878143.
Completed iteration 75 with error 0.0009045403638299199.
Completed iteration 100 with error 0.0006103613807980635.
Completed iteration 125 with error 0.0005311884300758241.
Completed iteration 150 with error 0.00043698527493063466.
Completed iteration 175 with error 0.00035198104401934494.
Completed iteration 200 with error 0.00028172941742177215.
Completed iteration 225 with error 0.0002251036430240827.
Completed iteration 250 with error 0.00017979635634568503.
Completed iteration 275 with error 0.0001436170545812132.
Completed iteration 300 with error 0.0001147362910276506.
Completed iteration 325 with error 9.167841288770795e-5.
Completed iteration 350 with error 7.326486227898776e-5.
Completed iteration 375 with error 5.855662116704963e-5.
Completed iteration 400 with error 4.680563708592267e-5.
Completed iteration 425 with error 3.741571258686349e-5.
Completed iteration 450 with error 2.9911412434691087e-5.
Completed iteration 475 with error 2.3913408442099993e-5.
Completed iteration 500 with error 1.9118921097849295e-5.
Completed iteration 525 with error 1.5286186529461787e-5.
Completed iteration 550 with error 1.2222105523429505e-5.
Completed iteration 575 with error 9.772412332020863e-6.
Completed iteration 600 with error 7.813842262560655e-6.
Completed iteration 625 with error 6.24788716541147e-6.
Completed iteration 650 with error 4.995813982278108e-6.
Completed iteration 675 with error 3.994689006692198e-6.
Completed iteration 700 with error 3.1942036058385526e-6.
Completed iteration 725 with error 2.554139072685757e-6.
Completed iteration 750 with error 2.042341417540783e-6.
Completed iteration 775 with error 1.63310321776855e-6.
Completed iteration 800 with error 1.3058705052859665e-6.
Completed iteration 825 with error 1.0442092637230616e-6.
Completed iteration 850 with error 8.349793416684292e-7.
Completed iteration 875 with error 6.676740960109839e-7.
Completed iteration 900 with error 5.338924871090001e-7.
Completed iteration 925 with error 4.2691703150410376e-7.
Completed iteration 950 with error 3.413763751680676e-7.
Completed iteration 975 with error 2.729755144148527e-7.
Completed iteration 1000 with error 2.1828008911484176e-7.
Completed iteration 1025 with error 1.7454391376681144e-7.
Completed iteration 1050 with error 1.3957107514173117e-7.
Completed iteration 1075 with error 1.1160566559809126e-7.
Completed iteration 1100 with error 8.924361205586706e-8.
Completed iteration 1125 with error 7.136217905490128e-8.
Completed iteration 1150 with error 5.706359385015958e-8.
Completed iteration 1175 with error 4.5629968914440155e-8.
Completed iteration 1200 with error 3.6487260768325314e-8.
Completed iteration 1225 with error 2.9176445481837732e-8.
Completed iteration 1250 with error 2.3330471332627667e-8.
Completed iteration 1275 with error 1.8655833988745485e-8.
Completed iteration 1300 with error 1.4917836299588316e-8.
Completed iteration 1325 with error 1.1928807275296549e-8.
Completed iteration 1350 with error 9.538678291676206e-9.
Completed iteration 1375 with error 7.627450449021467e-9.
Completed iteration 1400 with error 6.099168059492399e-9.
Completed iteration 1425 with error 4.877101611455714e-9.
Completed iteration 1450 with error 3.899895739678527e-9.
Completed iteration 1475 with error 3.1184890225688378e-9.
Completed iteration 1500 with error 2.4936497311500716e-9.
Completed iteration 1525 with error 1.9940069595492105e-9.
Completed iteration 1550 with error 1.594474996480244e-9.
Completed iteration 1575 with error 1.2749967748248991e-9.
Completed iteration 1600 with error 1.019530682100367e-9.
Completed iteration 1625 with error 8.152511998815726e-10.
Completed iteration 1650 with error 6.519025319562388e-10.
Completed iteration 1675 with error 5.212834608414596e-10.
Completed iteration 1700 with error 4.168356770861692e-10.
Completed iteration 1725 with error 3.3331604143427285e-10.
Completed iteration 1750 with error 2.6653124152176133e-10.
Completed iteration 1775 with error 2.1312729359124205e-10.
Completed iteration 1800 with error 1.704234531274551e-10.
Completed iteration 1825 with error 1.3627632355905916e-10.
Completed iteration 1850 with error 1.0897172053603299e-10.
Terminated successfully in 1861 iterations.
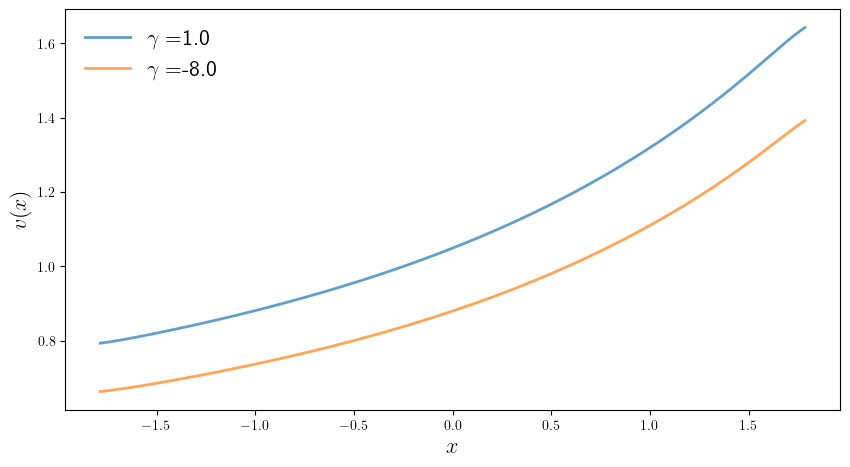
vary_alpha(savefig=true)
Completed iteration 25 with error 0.00705978038298638.
Completed iteration 50 with error 0.0016244720239244614.
Completed iteration 75 with error 0.0004469052464325385.
Completed iteration 100 with error 0.00019885551337017748.
Completed iteration 125 with error 0.0001136541590511797.
Completed iteration 150 with error 9.197108433633971e-5.
Completed iteration 175 with error 7.721086408851718e-5.
Completed iteration 200 with error 6.184474131387674e-5.
Completed iteration 225 with error 4.877743776932064e-5.
Completed iteration 250 with error 3.8269105249622015e-5.
Completed iteration 275 with error 2.9970764836795283e-5.
Completed iteration 300 with error 2.3457884571698173e-5.
Completed iteration 325 with error 1.835697143692805e-5.
Completed iteration 350 with error 1.4364647692444166e-5.
Completed iteration 375 with error 1.1240612030949393e-5.
Completed iteration 400 with error 8.796119907339417e-6.
Completed iteration 425 with error 6.883337746454998e-6.
Completed iteration 450 with error 5.386578291410871e-6.
Completed iteration 475 with error 4.215331874668493e-6.
Completed iteration 500 with error 3.298788607386527e-6.
Completed iteration 525 with error 2.581548538493905e-6.
Completed iteration 550 with error 2.020265916113928e-6.
Completed iteration 575 with error 1.581024815511256e-6.
Completed iteration 600 with error 1.2372866600163235e-6.
Completed iteration 625 with error 9.682848667313948e-7.
Completed iteration 650 with error 7.577690683824301e-7.
Completed iteration 675 with error 5.930227031658575e-7.
Completed iteration 700 with error 4.640943993550195e-7.
Completed iteration 725 with error 3.6319659924011205e-7.
Completed iteration 750 with error 2.8423500486596254e-7.
Completed iteration 775 with error 2.2244037589658205e-7.
Completed iteration 800 with error 1.7408040631217148e-7.
Completed iteration 825 with error 1.362342560984331e-7.
Completed iteration 850 with error 1.0661612370732598e-7.
Completed iteration 875 with error 8.343716673131496e-8.
Completed iteration 900 with error 6.529746099381839e-8.
Completed iteration 925 with error 5.1101434639377885e-8.
Completed iteration 950 with error 3.999170727908563e-8.
Completed iteration 975 with error 3.1297297642396416e-8.
Completed iteration 1000 with error 2.4493100214684205e-8.
Completed iteration 1025 with error 1.916817238267754e-8.
Completed iteration 1050 with error 1.5000912290119572e-8.
Completed iteration 1075 with error 1.1739636152086064e-8.
Completed iteration 1100 with error 9.187378857689055e-9.
Completed iteration 1125 with error 7.189995265832749e-9.
Completed iteration 1150 with error 5.626853871731896e-9.
Completed iteration 1175 with error 4.40354752662131e-9.
Completed iteration 1200 with error 3.4461931086582354e-9.
Completed iteration 1225 with error 2.6969735333892686e-9.
Completed iteration 1250 with error 2.1106374425983176e-9.
Completed iteration 1275 with error 1.651774494959568e-9.
Completed iteration 1300 with error 1.292670193109302e-9.
Completed iteration 1325 with error 1.011636996395282e-9.
Completed iteration 1350 with error 7.917018152170385e-10.
Completed iteration 1375 with error 6.195819413079562e-10.
Completed iteration 1400 with error 4.848825785330746e-10.
Completed iteration 1425 with error 3.794662362111012e-10.
Completed iteration 1450 with error 2.9696800574186e-10.
Completed iteration 1475 with error 2.3240565028004312e-10.
Completed iteration 1500 with error 1.8187962247395717e-10.
Completed iteration 1525 with error 1.4233747513969774e-10.
Completed iteration 1550 with error 1.1139311695274046e-10.
Terminated successfully in 1563 iterations.
Completed iteration 25 with error 0.007135885868511105.
Completed iteration 50 with error 0.0016055933560223945.
Completed iteration 75 with error 0.0004403182419771001.
Completed iteration 100 with error 0.00019170939378654328.
Completed iteration 125 with error 0.00010760706432144662.
Completed iteration 150 with error 7.947964446186617e-5.
Completed iteration 175 with error 6.72775698025152e-5.
Completed iteration 200 with error 5.4081885924706086e-5.
Completed iteration 225 with error 4.274895119582567e-5.
Completed iteration 250 with error 3.3598178110327837e-5.
Completed iteration 275 with error 2.6354627015390264e-5.
Completed iteration 300 with error 2.0659148304957853e-5.
Completed iteration 325 with error 1.6191113263008816e-5.
Completed iteration 350 with error 1.2688664558613283e-5.
Completed iteration 375 with error 9.943784911037312e-6.
Completed iteration 400 with error 7.792746775203696e-6.
Completed iteration 425 with error 6.107082487050164e-6.
Completed iteration 450 with error 4.786093088071652e-6.
Completed iteration 475 with error 3.7508697376953393e-6.
Completed iteration 500 with error 2.939582415262265e-6.
Completed iteration 525 with error 2.303782837165258e-6.
Completed iteration 550 with error 1.8055071036027215e-6.
Completed iteration 575 with error 1.415005903782074e-6.
Completed iteration 600 with error 1.1089664067043259e-6.
Completed iteration 625 with error 8.69119277879804e-7.
Completed iteration 650 with error 6.811473081125285e-7.
Completed iteration 675 with error 5.338303132873534e-7.
Completed iteration 700 with error 4.1837509945352735e-7.
Completed iteration 725 with error 3.278904350256795e-7.
Completed iteration 750 with error 2.569756183401495e-7.
Completed iteration 775 with error 2.013980631154766e-7.
Completed iteration 800 with error 1.5784063345236632e-7.
Completed iteration 825 with error 1.237036351131593e-7.
Completed iteration 850 with error 9.694964009376861e-8.
Completed iteration 875 with error 7.598187723445449e-8.
Completed iteration 900 with error 5.954892090542785e-8.
Completed iteration 925 with error 4.6670001196957855e-8.
Completed iteration 950 with error 3.657646874266618e-8.
Completed iteration 975 with error 2.8665911644409903e-8.
Completed iteration 1000 with error 2.2466207783011782e-8.
Completed iteration 1025 with error 1.760734136269093e-8.
Completed iteration 1050 with error 1.3799324571905913e-8.
Completed iteration 1075 with error 1.0814884321419527e-8.
Completed iteration 1100 with error 8.47590220232064e-9.
Completed iteration 1125 with error 6.6427816580727495e-9.
Completed iteration 1150 with error 5.2061188693386384e-9.
Completed iteration 1175 with error 4.080168647391247e-9.
Completed iteration 1200 with error 3.1977331893529026e-9.
Completed iteration 1225 with error 2.5061452912922277e-9.
Completed iteration 1250 with error 1.9641301918227327e-9.
Completed iteration 1275 with error 1.5393402108543341e-9.
Completed iteration 1300 with error 1.2064198529060377e-9.
Completed iteration 1325 with error 9.45502121041386e-10.
Completed iteration 1350 with error 7.41014583027777e-10.
Completed iteration 1375 with error 5.807518910216913e-10.
Completed iteration 1400 with error 4.551501397997981e-10.
Completed iteration 1425 with error 3.567128814552234e-10.
Completed iteration 1450 with error 2.7956503778625574e-10.
Completed iteration 1475 with error 2.191020698205648e-10.
Completed iteration 1500 with error 1.717159747727237e-10.
Completed iteration 1525 with error 1.3457812642059253e-10.
Completed iteration 1550 with error 1.0547274165162435e-10.
Terminated successfully in 1557 iterations.
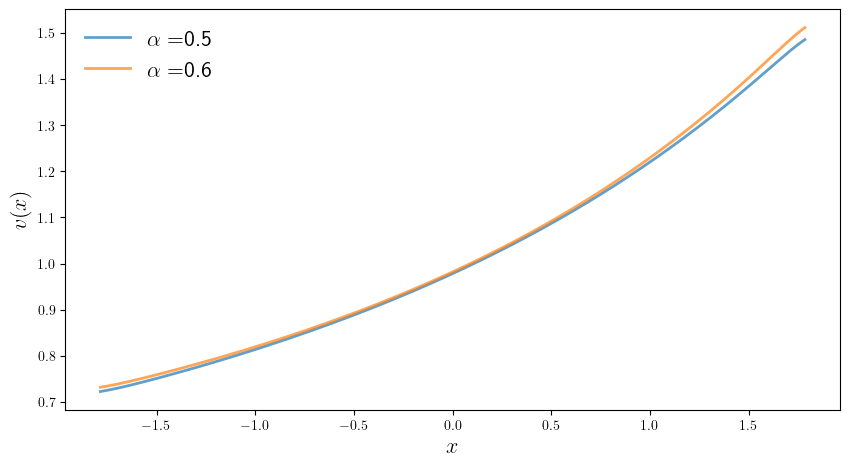